- Published on
π Simple Clean Code Rules for Every Developer
- Authors
- Name
- Wisit Longsida
- @__ART3MISS
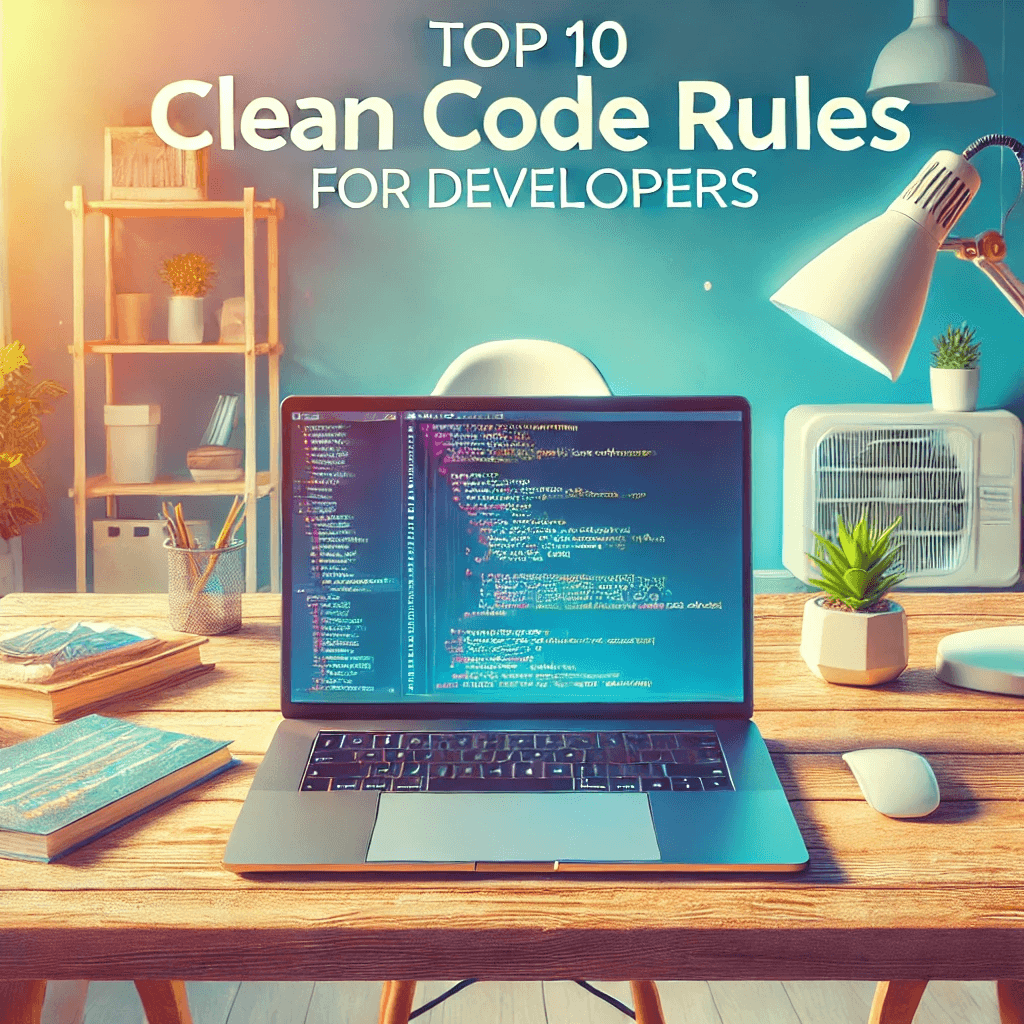
Clean code makes your programs easy to read, understand, and update. Follow these rules to write better code that you and others can work with easily!
1. Use Clear and Meaningful Names
Give variables, functions, and classes meaningful names that explain their purpose.
β Bad:
a = 10
β Good:
max_retries = 10
π Why? A good name tells you what the code does without needing extra comments.
2. Keep Functions Small and Focused
Each function should do one thing only. If it does too many things, it gets hard to read and debug.
β Bad:
def process_order(order):
validate_order(order)
calculate_total(order)
apply_discount(order)
ship_order(order)
β Good:
def validate_order(order):
pass
def calculate_total(order):
pass
def apply_discount(order):
pass
π Why? Small functions are easier to understand and test.
3. Avoid Deep Nesting
Too many levels of nesting make code hard to follow.
β Bad:
if user:
if user.is_active:
if order:
process_order(order)
β Good:
if not user or not user.is_active:
return
if not order:
return
process_order(order)
π Why? Exiting early keeps your code simple and clear.
4. Use Comments Wisely
Write comments to explain "why," not "what." Good code should explain itself.
β Bad:
# Set the user as active
user.is_active = True
β Good:
# Activate the user after successful login
user.is_active = True
π Why? Comments should add value by explaining reasons or business logic.
5. Use Consistent Formatting
Keep your code neat with consistent indentation, spacing, and alignment.
β Bad:
def add(a,b):return a+b
β Good:
def add(a, b):
return a + b
π Why? Neat code is easier to read and maintain.
6. Donβt Repeat Yourself (DRY)
Avoid duplicating the same code in different places.
β Bad:
if user_type == "admin":
# complex logic
if user_type == "superadmin":
# same complex logic
β Good:
def user_is_admin(user_type):
return user_type in ["admin", "superadmin"]
if user_is_admin(user_type):
# complex logic
π Why? Reusing code reduces errors and makes updates easier.
7. Follow Single Responsibility Principle (SRP)
A class or function should have only one job.
β Bad:
class User:
def register(self):
pass
def login(self):
pass
def send_email(self):
pass
β Good:
class User:
def register(self):
pass
def login(self):
pass
class EmailService:
def send_email(self):
pass
π Why? Breaking responsibilities into smaller parts makes your code more modular.
8. Avoid Magic Numbers and Strings
Use constants instead of hardcoding values directly in your code.
β Bad:
discount = 0.05
if user.role == "admin":
pass
β Good:
DISCOUNT_RATE = 0.05
ADMIN_ROLE = "admin"
discount = DISCOUNT_RATE
if user.role == ADMIN_ROLE:
pass
π Why? Constants make your code easier to understand and change.
9. Write Tests
Tests make sure your code works and helps prevent bugs when making changes.
β Bad:
def process_order(order):
pass # no tests
β Good:
def test_process_order():
order = create_test_order()
result = process_order(order)
assert result == expected_result
π Why? Testing keeps your code stable and reliable.
π Keep It Simple (KISS)
Simplicity is key. Avoid overcomplicating things.
β Bad:
def add(a, b):
if isinstance(a, int) and isinstance(b, int):
return a + b
β Good:
def add(a, b):
return a + b
π Why? Simple code is easier to read and maintain.
β¨ Summary:
Writing clean code isn't just about making it workβit's about making it easy to understand, update, and improve. Follow these 10 simple rules, and your code will stand out! π